1. Java ํ๋ก์ ํธ MySQL ์ฐ๋
- JDBC ๋๋ผ์ด๋ฒ ์ค์น : https://dev.mysql.com/downloads/connector/j/
MySQL :: Download Connector/J
MySQL Connector/J 8.0 is highly recommended for use with MySQL Server 8.0, 5.7 and 5.6. Please upgrade to MySQL Connector/J 8.0.
dev.mysql.com
2. JDBC ํ์ผ ์์ถ ํด์ ํ Java ํ๋ก์ ํธ ์ฐํด๋ฆญ - Properties - Java Build Path - Libraries ์ mysql-connector-java-๋ฒ์ .jar ์ถ๊ฐ
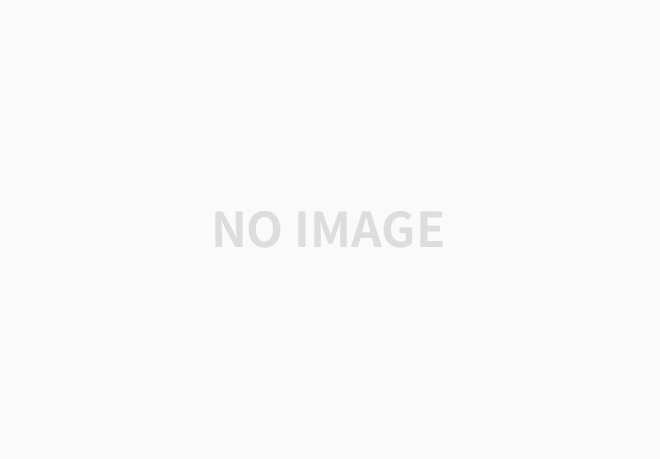
3. Java ํ๋ก์ ํธ ์คํ
2. CRUD ํ๋ก๊ทธ๋จ ์์ฑ (Insert, Select, Update, Delete)
DB๋ช : DB01
ํ ์ด๋ธ๋ช : TABLE01
VALUE | TYPE |
fullname | varchar |
age | int |
salary | int |
1. ์ซ์๋ฅผ ์ ๋ ฅํ๋ฉด ๊ฐ ์ซ์์ ๋ง๋ ํจ์๊ฐ ์คํ๋๋๋ก ํ๋ก๊ทธ๋จ ์์ฑ
package testdb;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.ResultSet;
import java.sql.Statement;
import java.util.Scanner;
import java.sql.SQLException;
import java.sql.PreparedStatement;
public class Main {
static String jdbc_driver = "com.mysql.cj.jdbc.Driver";
static String jdbc_url = "jdbc:mysql://localhost:3306/DB01";
public static void main(String[] args) throws ClassNotFoundException {
while(true) {
System.out.print("์ซ์๋ฅผ ์
๋ ฅํ์ธ์ : ");
Scanner scanner = new Scanner(System.in);
int n = scanner.nextInt();
System.out.println(n);
if(n==5) {
System.out.println("์ข
๋ฃ๋์์ต๋๋ค.");
break;
}
switch(n) {
case 1:
insert();
break;
case 2:
select();
break;
case 3:
update();
break;
case 4:
delete();
break;
default:
break;
}
}
}
}
2. INSERT
public static void insert() {
Connection con = null;
PreparedStatement pstmt = null;
System.out.println("Insert ์ฟผ๋ฆฌ");
try {
Class.forName(jdbc_driver);
con = DriverManager.getConnection(jdbc_url, "root", "1234");
String sql = "INSERT INTO TABLE01 VALUES (?,?,?)";
pstmt = con.prepareStatement(sql);
pstmt.setString(1, "ํ๋ง");
pstmt.setInt(2, 10);
pstmt.setInt(3, 2000);
pstmt.executeUpdate();
int count = pstmt.executeUpdate();
if(count == 0){
System.out.println("์
๋ ฅ ์คํจ");
}
else{
System.out.println("์
๋ ฅ ์ฑ๊ณต");
}
}
catch( ClassNotFoundException e){
System.out.println("์ค๋ฅ");
}
catch( SQLException e){
System.out.println("์ค๋ฅ" + e);
}
finally{
try{
if(con != null && !con.isClosed()){
con.close();
}
if(pstmt != null && !pstmt.isClosed()){
pstmt.close();
}
}
catch( SQLException e){
e.printStackTrace();
}
}
}
- Connection : ๋ฐ์ดํฐ๋ฒ ์ด์ค์ ์ฐ๊ฒฐํ๋ ๊ฐ์ฒด
- DriverManager : JDBC ๋๋ผ์ด๋ฒ๋ฅผ ํตํด Connection์ ๋ง๋๋ ์ญํ
- PreparedStatement : SQL ์ฟผ๋ฆฌ๋ฌธ์ ๋ฐ์ดํฐ๋ฒ ์ด์ค์ ๋ณด๋ด๊ธฐ ์ํ ๊ฐ์ฒด
- Statement : SQL ์ฟผ๋ฆฌ๋ฌธ์ ๋ฐ์ดํฐ๋ฒ ์ด์ค์ ๋ณด๋ด๊ธฐ ์ํ ๊ฐ์ฒด
PreparedStatement | Statement |
- SQL ์ฟผ๋ฆฌ๋ฌธ์ ๋ฐ์ดํฐ๋ฒ ์ด์ค์ ๋ณด๋ด๊ธฐ ์ํ ๊ฐ์ฒด - try catch ๋ฌธ์ผ๋ก ์์ธ ์ฒ๋ฆฌํด์ค์ผ ํจ |
|
- ๋์ ์ธ ์ฟผ๋ฆฌ๋ฌธ์ ์ ํฉ - ๊ฐ ๋ณ๊ฒฝํ๊ฑฐ๋ ํ๋ผ๋ฏธํฐ๊ฐ ๋ง์ ๊ฒฝ์ฐ์ ์ ํฉ - INSERT, UPDATE, DELETE |
- ์ ์ ์ธ ์ฟผ๋ฆฌ๋ฌธ์ ์ ํฉ - SELECT |
3. SELECT
public static void select() throws ClassNotFoundException {
Connection con = null;
Statement stmt = null;
ResultSet rs = null;
System.out.println("Select ์ฟผ๋ฆฌ");
try {
Class.forName(jdbc_driver);
con = DriverManager.getConnection(jdbc_url, "root", "1234");
String sql = "SELECT * FROM TABLE01";
stmt = con.createStatement();
rs = stmt.executeQuery(sql);
while(rs.next()) {
String name = rs.getString("FULLNAME");
int age = rs.getInt("AGE");
int salary = rs.getInt("SALARY");
System.out.println(name + " " + age + " " + salary);
}
}
catch (SQLException e) {
System.out.println("์ค๋ฅ : " + e.getMessage());
}
catch (ClassNotFoundException e1) {
System.out.println("์ค๋ฅ : " + e1.getMessage());
}
finally {
if(rs != null) {
try {
rs.close();
} catch (Exception e) {
e.printStackTrace();
}
}
if(stmt != null) {
try {
stmt.close();
} catch (Exception e) {
e.printStackTrace();
}
}
if(con != null) {
try {
con.close();
} catch (Exception e) {
e.printStackTrace();
}
}
}
}
4. UPDATE
public static void update() {
Connection con = null;
PreparedStatement pstmt = null;
System.out.println("Update ์ฟผ๋ฆฌ");
try {
Class.forName(jdbc_driver);
con = DriverManager.getConnection(jdbc_url, "root", "1234");
String sql = "UPDATE TABLE01 SET FULLNAME=?, AGE=? WHERE SALARY=?";
pstmt = con.prepareStatement(sql);
pstmt.setString(1, "๋ฉ๋๊ธฐ");
pstmt.setInt(2, 20);
pstmt.setInt(3, 4000);
int count = pstmt.executeUpdate();
System.out.println(pstmt);
System.out.println("๋ณ๊ฒฝ๋ row : " + count);
}
catch (SQLException e) {
System.out.println("์ค๋ฅ : " + e.getMessage());
}
catch (ClassNotFoundException e1) {
System.out.println("์ค๋ฅ : " + e1.getMessage());
}
finally {
if(pstmt != null) {
try {
pstmt.close();
} catch (Exception e) {
e.printStackTrace();
}
}
if(con != null) {
try {
con.close();
} catch (Exception e) {
e.printStackTrace();
}
}
}
}
5. DELETE
public static void delete() {
Connection con = null;
PreparedStatement pstmt = null;
System.out.println("Delete ์ฟผ๋ฆฌ");
try {
Class.forName(jdbc_driver);
con = DriverManager.getConnection(jdbc_url, "root", "1234");
String sql = "DELETE FROM TABLE01 WHERE FULLNAME=?";
pstmt = con.prepareStatement(sql);
pstmt.setString(1, "๋ฉ๋๊ธฐ");
int count = pstmt.executeUpdate();
System.out.println(pstmt);
System.out.println("๋ณ๊ฒฝ๋ row : " + count);
}
catch (SQLException e) {
System.out.println("์ค๋ฅ : " + e.getMessage());
}
catch (ClassNotFoundException e1) {
System.out.println("์ค๋ฅ : " + e1.getMessage());
}
finally {
if(pstmt != null) {
try {
pstmt.close();
} catch (Exception e) {
e.printStackTrace();
}
}
if(con != null) {
try {
con.close();
} catch (Exception e) {
e.printStackTrace();
}
}
}
}
'Database' ์นดํ ๊ณ ๋ฆฌ์ ๋ค๋ฅธ ๊ธ
PostgreSQL ์ฌ์ฉํ๊ธฐ (0) | 2021.09.15 |
---|
๋๊ธ